기존에 있던 lib 업로드
1. repository 생성
- nexus에 로그인 후, create repository 클릭
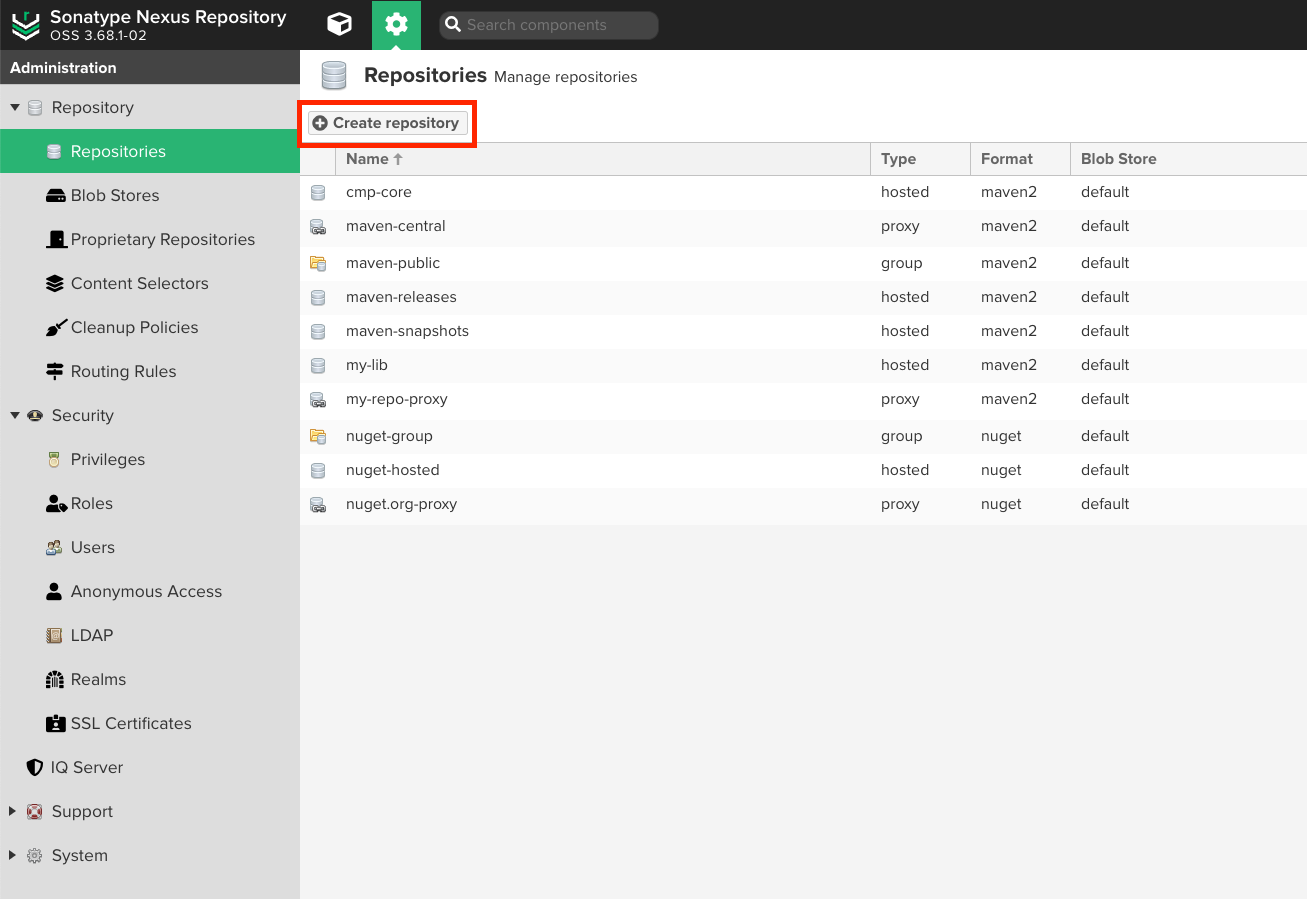
- maven2 (hosted) 클릭
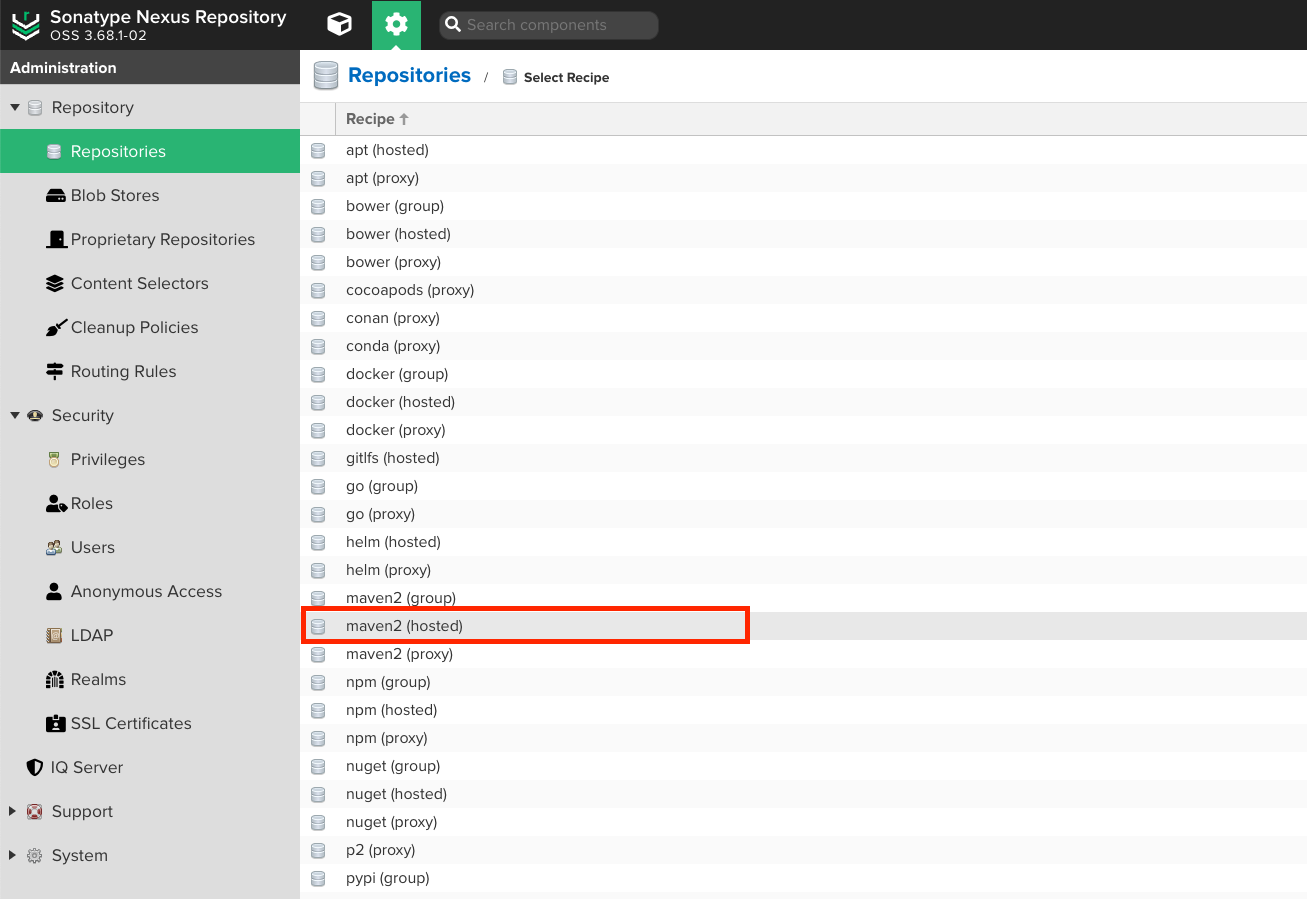
- 새로 만들 repository 내용 작성
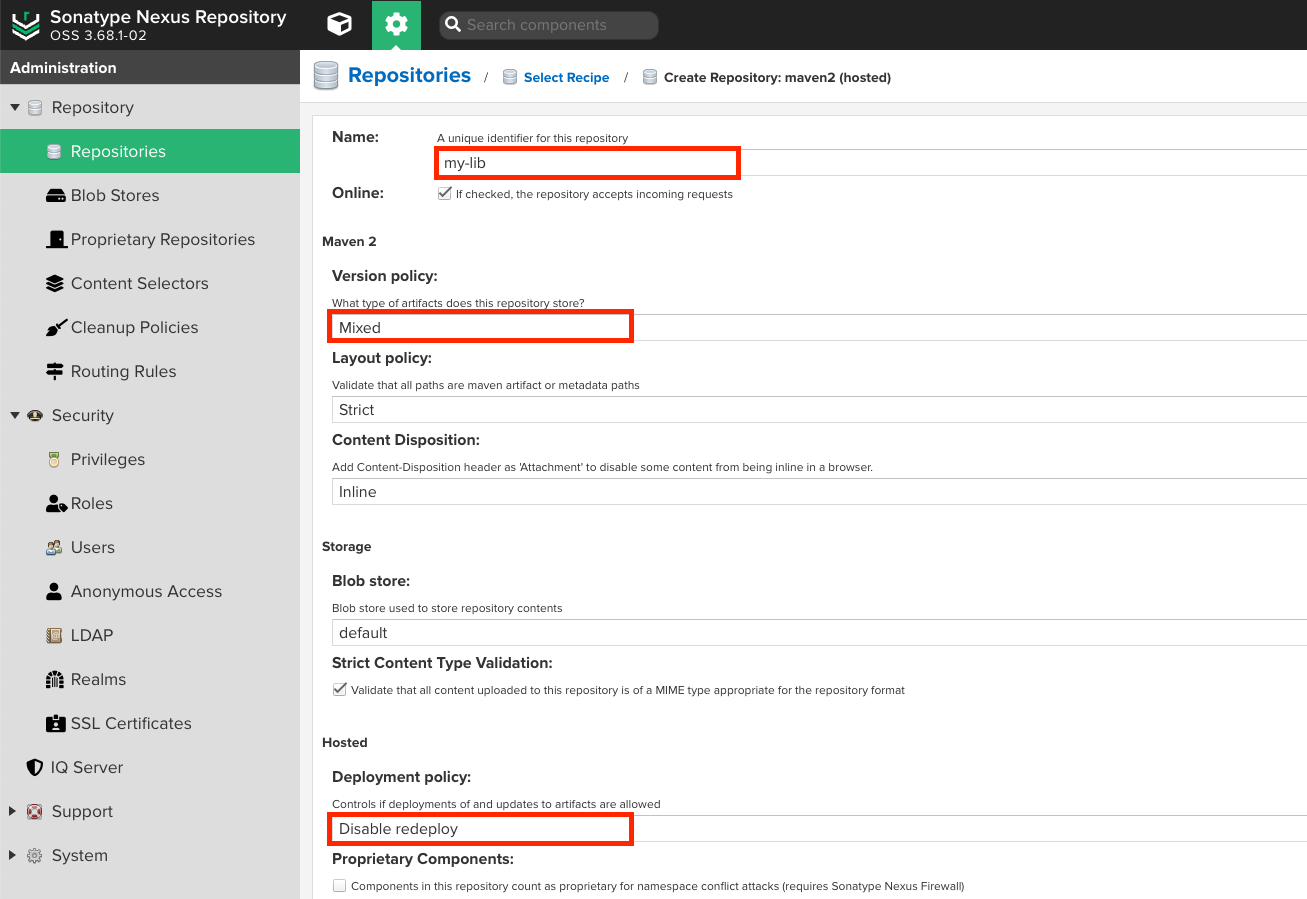
- Name: 새로 만들 repository 이름
- Version policy: 어떤 타입의 repository를 만들 것인가
- Deployment policy: 배포 방법

- 다 기입 후 Create repository 클릭
2. Repository access Role 생성
- Roles > Create Role 클릭

- Role 내용 작성

- Type: Nexus role
- Role ID: Role ID
- Role Name: Role Name
3가지 작성 후, Modify Applied Privileges 클릭
- 아까 만든 repository 명으로 검색, nx-repository-xx-my-lib-* 처럼 아까만든 레포지터리로 된 권한 다 주고,

- nx-component-upload 도 추가해주고 하단의 save 클릭
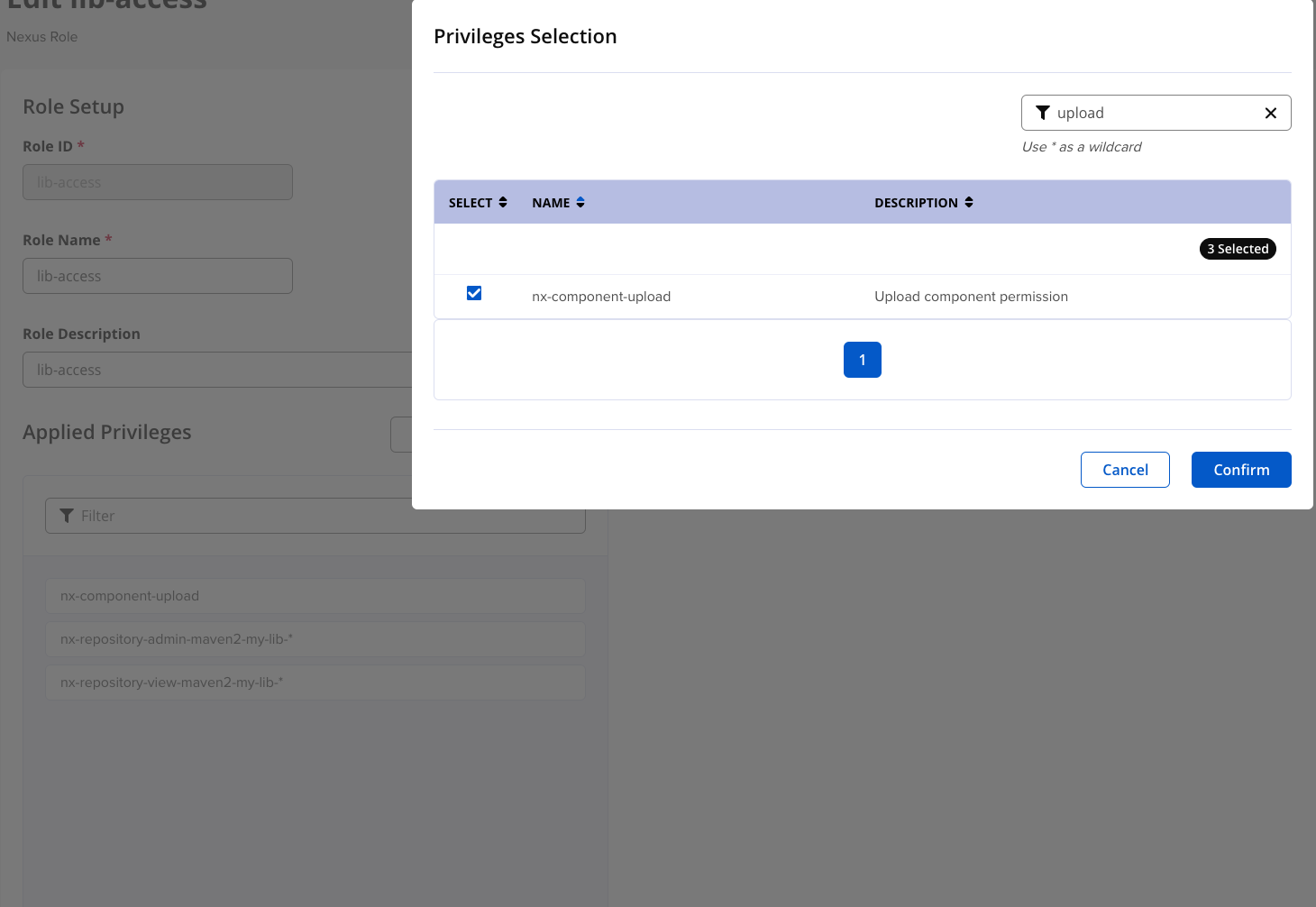
3. User 생성
- Users > Create local user 클릭
- User 정보 작성
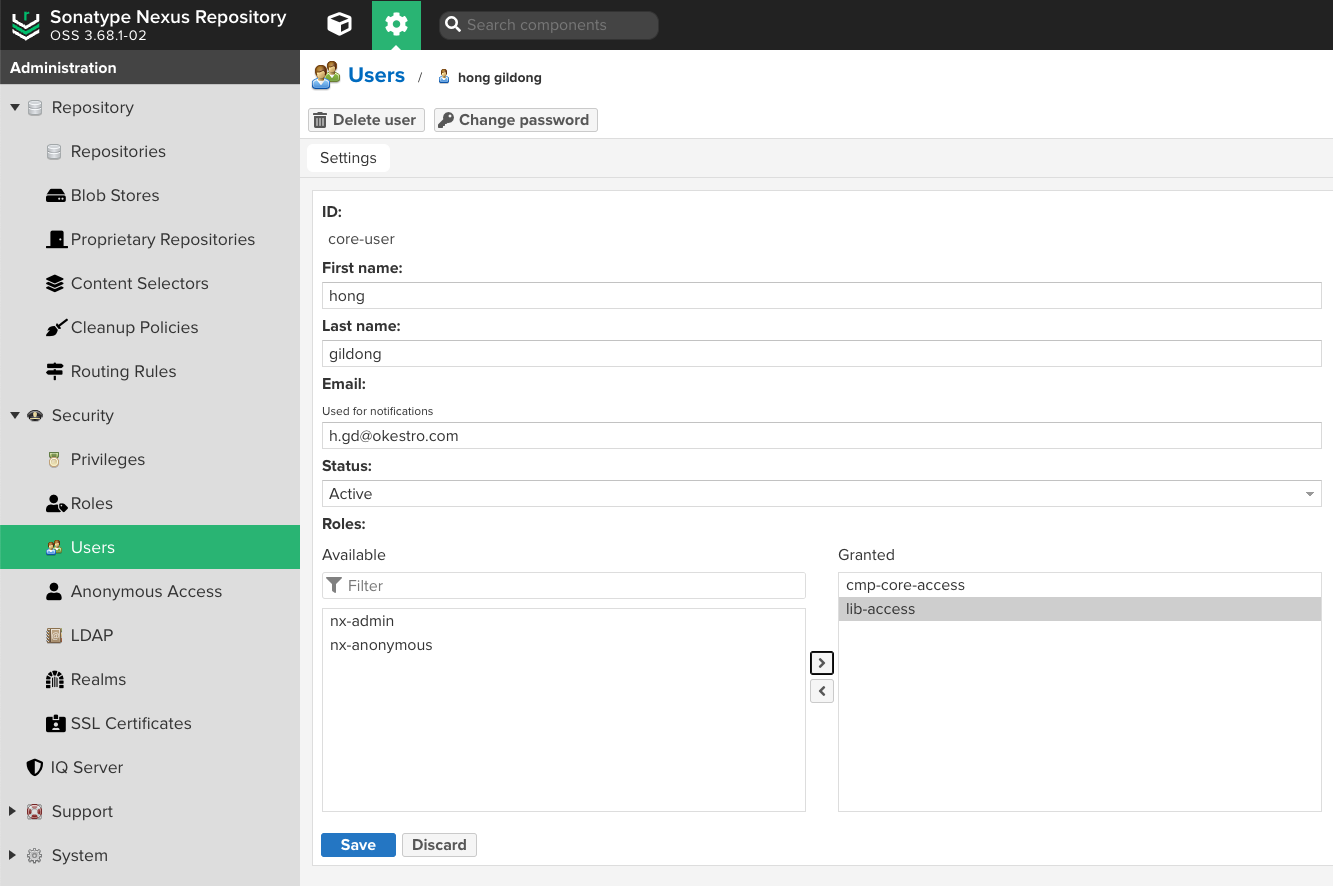
- 작성할 때 아까 만든 Role을 Granted 해주고 save
4. 라이브러리 업로드
- settings.xml 설정
nexus 레파지토리에 접근하기 위해서는 우선, maven 설정 파일인 settings.xml을 수정해야야 한다. 기본적으로는 ~/.m2/settings.xml 으로 설정되어 있으므로 이 곳에 생성해서 사용하거나, 이미 settings.xml을 사용하고 있다면 그 파일을 수정해주면 되는데.. 이도 저도 모르겠으면 아래 이미지 처럼 인텔리제이에서 확인하여 그 위치의 settings.xml을 수정해준다.
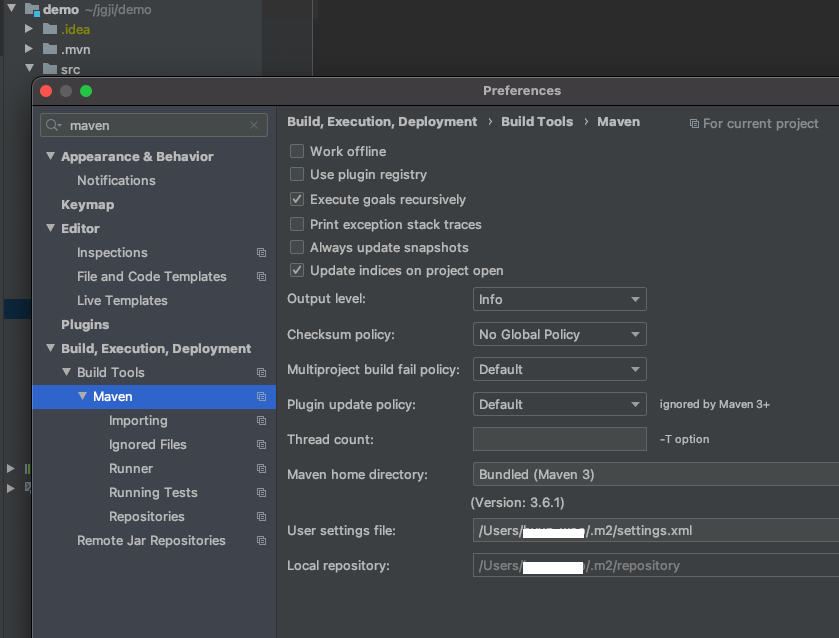
<settings xmlns="http://maven.apache.org/SETTINGS/1.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/SETTINGS/1.0.0
http://maven.apache.org/xsd/settings-1.0.0.xsd">
<servers>
<server>
<id>nexus</id>
<username>core-user</username>
<password>admin123!</password>
</server>
<server>
<id>my-lib</id> # repository 이름
<username>admin</username> # repository에 접근 권한이 있는 유저 이름
<password>admin123!</password> # repository에 접근 권한이 있는 유저 비밀번호
</server>
</servers>
</settings>
- 라이브러리 nexus에 업로드
cp /Users/gtpark/.m2/repository/org/springframework/boot/spring-boot-starter-web/2.6.6/spring-boot-starter-web-2.6.6.jar /Users/gtpark/temp/spring-boot-starter-web-2.6.6.jar
mvn deploy:deploy-file \
-DgroupId=org.springframework.boot \
-DartifactId=spring-boot-starter-web \
-Dversion=2.6.6 \
-Dpackaging=jar \
-Dfile=/Users/gtpark/temp/spring-boot-starter-web-2.6.6.jar \
-DrepositoryId=nexus \
-Durl=http://nexus.repository.com/repository/cmp-core/ \
-X
- nexus에서 확인
Browse > 확인할 repository 클릭
라이브러리가 잘 올라갔는지 확인 완료

5. 업로드 된 라이브러리 사용하기
새로 만든 프로젝트에 pom.xml 을 설정해준다.
- pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.6</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>bravo-api</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>bravo-api</name>
<description>bravo-api</description>
<properties>
<java.version>1.8</java.version>
</properties>
<repositories>
<repository>
<id>nexus</id>
<url>http://nexus.repository.com/repository/core/</url>
</repository>
<repository>
<id>my-lib</id>
<url>http://nexus.repository.com/repository/my-lib/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>com.util</groupId>
<artifactId>util-library</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.6.6</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>2.5.2</version>
</plugin>
</plugins>
</build>
</project>
custom lib 업로드
위의 1~3을 똑같이 해준다.
4. 라이브러리 업로드
settings.xml 까지 똑같이 위와 같이 세팅해준다.
다른 프로젝트에서 사용할 코드를 작성한다.
ex) DateTimeUtils
package com.util.utils;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
import java.time.temporal.ChronoUnit;
public class DateTimeUtils {
// Format a LocalDate to a string
public static String formatDate(LocalDate date, String pattern) {
if (date == null || pattern == null) {
return null;
}
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(pattern);
return date.format(formatter);
}
// Parse a string to a LocalDate
public static LocalDate parseDate(String dateStr, String pattern) {
if (dateStr == null || pattern == null) {
return null;
}
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(pattern);
return LocalDate.parse(dateStr, formatter);
}
// Get the current date and time
public static LocalDateTime getCurrentDateTime() {
return LocalDateTime.now();
}
// Get the difference in days between two LocalDates
public static long getDaysBetween(LocalDate startDate, LocalDate endDate) {
if (startDate == null || endDate == null) {
return 0;
}
return ChronoUnit.DAYS.between(startDate, endDate);
}
// Add days to a LocalDate
public static LocalDate addDays(LocalDate date, int days) {
if (date == null) {
return null;
}
return date.plusDays(days);
}
// Get the current time
public static LocalTime getCurrentTime() {
return LocalTime.now();
}
// Format a LocalDateTime to a string
public static String formatDateTime(LocalDateTime dateTime, String pattern) {
if (dateTime == null || pattern == null) {
return null;
}
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(pattern);
return dateTime.format(formatter);
}
// Parse a string to a LocalDateTime
public static LocalDateTime parseDateTime(String dateTimeStr, String pattern) {
if (dateTimeStr == null || pattern == null) {
return null;
}
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(pattern);
return LocalDateTime.parse(dateTimeStr, formatter);
}
}
- 라이브러리용 pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.util</groupId>
<artifactId>util-library</artifactId>
<version>1.0.0</version>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
</properties>
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<version>3.2.0</version>
<configuration>
<archive>
<manifestEntries>
<Export-Package>com.util.common</Export-Package>
<Import-Package>com.util.common</Import-Package>
</manifestEntries>
</archive>
<finalName>Util Library</finalName>
</configuration>
</plugin>
</plugins>
</build>
<!-- 사설 레포지터리 정보 -->
<distributionManagement>
<repository>
<id>nexus</id>
<url>http://nexus.repository.com/repository/core/</url>
</repository>
</distributionManagement>
<!-- build 명령어: mvn clean compile deploy -->
</project>
다음 명령어를 실행한다.
mvn clean compile deploy
** 주의 : intelliJ 자체 내장 mvn 버전이랑 호환이 안될수도 있으니 자신의 pc에 maven을 설치해서 terminal로 실행시켜주자.
nexus에서 라이브러리가 잘 업로드 됐는지 확인해주면 된다.
5. 업로드 된 라이브러리 사용하기
새로 만든 프로젝트에 pom.xml 을 설정해준다.
dependency 내용은
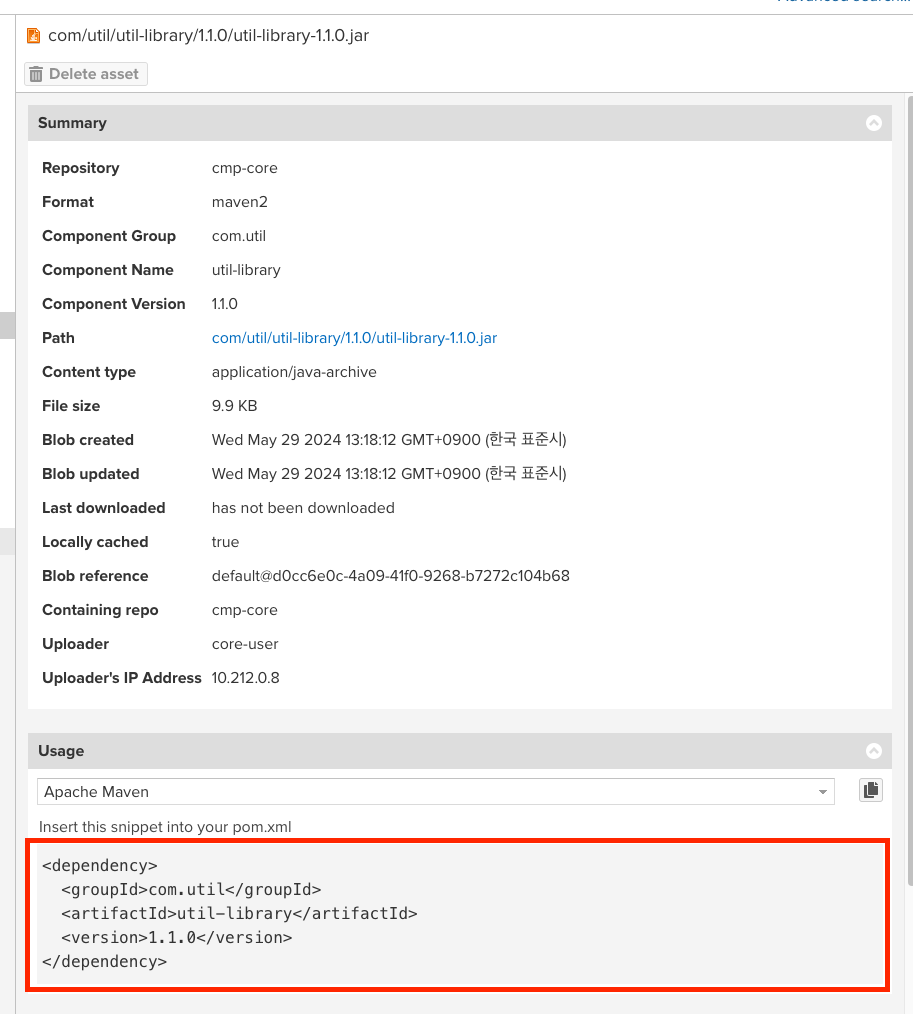
참조하면 된다.
- 새로 만든 프로젝트의 pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.6.6</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.example</groupId>
<artifactId>example-api</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>example-api</name>
<description>example-api</description>
<properties>
<java.version>1.8</java.version>
</properties>
<repositories>
<repository>
<id>nexus</id>
<url>http://nexus.repository.com/repository/core/</url>
</repository>
<repository>
<id>my-lib</id>
<url>http://nexus.repository.com/repository/my-lib/</url>
</repository>
</repositories>
<dependencies>
<dependency>
<groupId>com.util</groupId>
<artifactId>util-library</artifactId>
<version>1.0.0</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
<version>2.6.6</version>
</dependency>
<dependency>
<groupId>org.hibernate</groupId>
<artifactId>hibernate-core</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>2.5.2</version>
</plugin>
</plugins>
</build>
</project>
그럼 이제 다음과 같이 implement한 라이브러리 내용을 사용할 수 있다.
package com.example.bravoapi.controller;
import com.util.utils.StringUtils;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@GetMapping("/hello")
public String hello() {
return "hello";
}
@GetMapping("/str/{str}")
public String reverse (@PathVariable String str) {
return StringUtils.reverse(str);
}
}
(**) gradle의 경우
gradle 프로젝트의 경우 settings.xml을 따로 설정할 필요없이 build.gradle에서 간단히 설정이 가능하다.
- build.gradle
plugins {
id 'maven-publish'
}
group = 'com.yourcompany'
version = '1.0.0-SNAPSHOT'
publishing {
publications {
mavenJava(MavenPublication) {
from components.java
}
}
repositories {
maven {
name = "nexus"
url = uri("http://your-nexus-repo-url/repository/maven-releases/")
credentials {
username = project.findProperty("nexusUsername") ?: "user"
password = project.findProperty("nexusPassword") ?: "password"
}
}
}
}
- gradle.properties
nexusUsername=admin
nexusPassword=adminPassword
다음 명령어를 실행해준다.
./gradlew clean build publish
사용하는 쪽은
- build.gradle
repositories {
mavenCentral()
maven {
url "http://your-nexus-repo-url/repository/maven-releases/"
}
}
dependencies {
implementation 'com.yourcompany:core-common:1.0.0-SNAPSHOT'
implementation 'com.yourcompany:core-persistence:1.0.0-SNAPSHOT'
implementation 'com.yourcompany:core-service:1.0.0-SNAPSHOT'
implementation 'org.springframework.boot:spring-boot-starter-web'
}
이런식으로 해주면 된다.
- script로 nexsus에 라이브러리 푸쉬하는 방법이 나와있다.
https://github.com/sonatype-nexus-community/nexus-repository-import-scripts/tree/master
GitHub - sonatype-nexus-community/nexus-repository-import-scripts: A few scripts for importing artifacts into Nexus Repository
A few scripts for importing artifacts into Nexus Repository - sonatype-nexus-community/nexus-repository-import-scripts
github.com
- 프로젝트에 있는 dependency jar 로 빼는 방법
https://devbrain.tistory.com/107
jar 파일 의존성 한번에 다운로드 maven 사용
먼저 mvn 명령이 cmd에서 실행될 수 있도록 다운로드 및 path에 등록되어 있어야 함 라이브러리를 다운로드 할 폴더를 생성 mkdir d:/jar_download 다운로드 할 jar 파일 검색 : mvnrepository.com/ 테스트로 대
devbrain.tistory.com
'미들웨어' 카테고리의 다른 글
Jenkins - Pipeline 형식 (0) | 2024.12.15 |
---|---|
Docker Image 폐쇄망 옮기기 (0) | 2024.11.30 |